Table of Contents
NPM initialization
npm init
This utility will walk you through creating a package.json file.
It only covers the most common items, and tries to guess sensible defaults.
See `npm help init` for definitive documentation on these fields
and exactly what they do.
Use `npm install ` afterwards to install a package and
save it as a dependency in the package.json file.
Press ^C at any time to quit.
package name: (2.3-npm) learning-npm
version: (1.0.0)
description: I'm learning about NPM
entry point: (index.js)
test command:
git repository:
keywords:
author: Hoomaan
license: (ISC)
About to write to /Users/xxx/Node.js/2.3 NPM/package.json:
{
"name": "learning-npm",
"version": "1.0.0",
"description": "I'm learning about NPM",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "Hoomaan",
"license": "ISC"
}
Is this OK? (yes) y
Instal NPM Packages
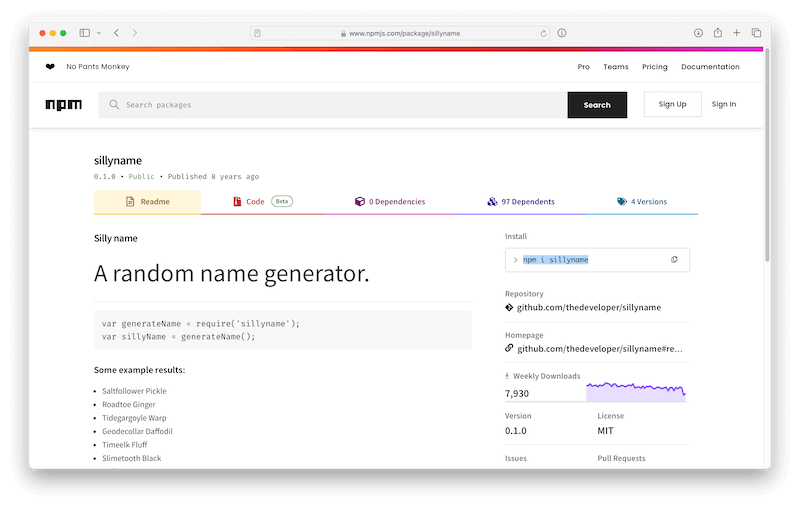
main.tsx
npm i sillyname
Issue:
In case of facing issue by running the command use the solution on line 16 of the following commands and try again:
npm i sillyname
npm ERR! code EACCES
npm ERR! syscall open
npm ERR! path /Users/xxx/.npm/_cacache/tmp/3d31c56f
npm ERR! errno EACCES
npm ERR!
npm ERR! Your cache folder contains root-owned files, due to a bug in
npm ERR! previous versions of npm which has since been addressed.
npm ERR!
npm ERR! To permanently fix this problem, please run:
npm ERR! sudo chown -R 501:20 "/Users/xxx/.npm"
npm ERR! A complete log of this run can be found in: /Users/xxx/.npm/_logs/2024-01-07T09_59_24_625Z-debug-0.log
sudo npm cache clean --force
npm WARN using --force Recommended protections disabled.
npm i sillyname
added 1 package, and audited 2 packages in 718ms
found 0 vulnerabilities
After successfully installation the package you will see a new section in your package.json file called “dependencies” and the name of the package (in this case: “sillyname”) inside it.
{
"name": "learning-npm",
"version": "1.0.0",
"description": "I'm learning about NPM",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "Hoomaan",
"license": "ISC",
"dependencies": {
"sillyname": "^0.1.0"
}
}
var generateName = require('sillyname');
var sillyName = generateName();
console.log(`My name is ${sillyName}.`);
ECMAScript
In case you want to use ECMAScript type, you need to update the package.json file and declare the type in it:
{
"name": "learning-npm",
"version": "1.0.0",
"description": "I'm learning about NPM",
"main": "index.js",
"type": "module",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "Hoomaan",
"license": "ISC",
"dependencies": {
"sillyname": "^0.1.0"
}
}
// var generateName = require('sillyname');
import generateName from 'sillyname';
var sillyName = generateName();
console.log(`My name is ${sillyName}.`);